What are JavaScript Functions? Explained For Beginners
September 1, 2022 β’ 0 minute read β’
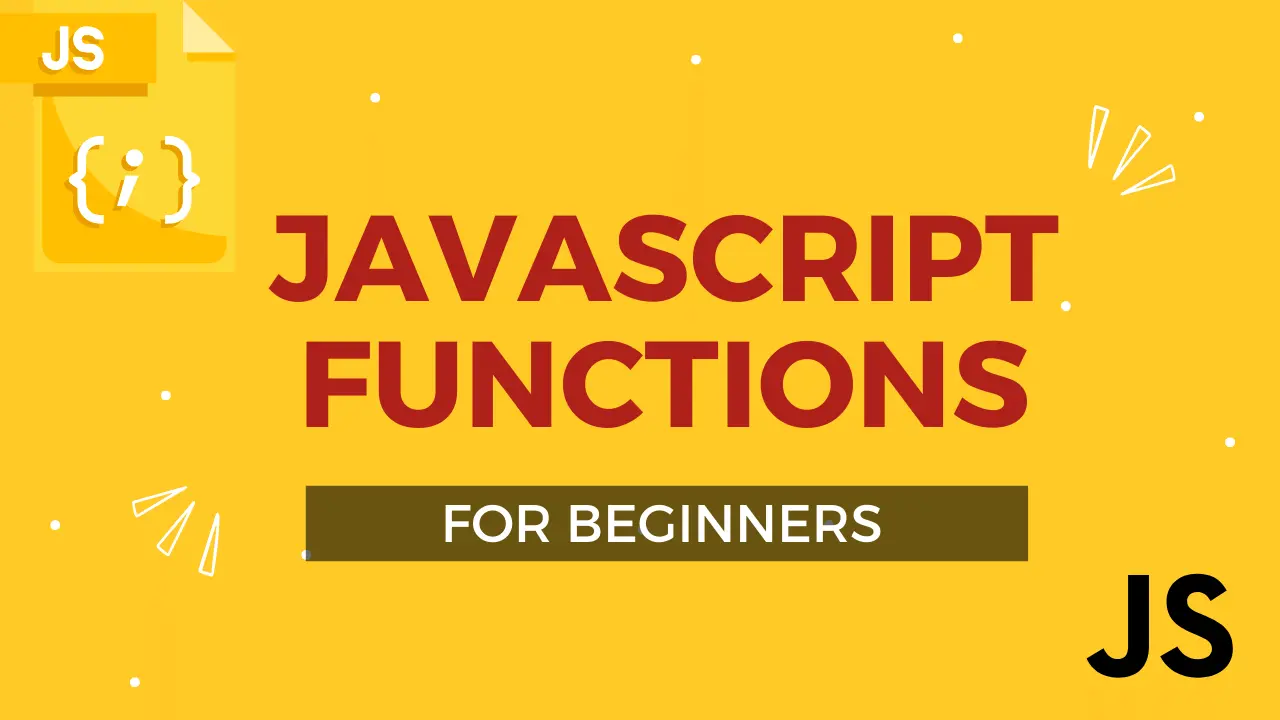
If you've used console.log()
in your JavaScript code, then you've worked with functions.
The console.log()
statement contains several lines of code you don't see, and this is what actually tells the JavaScript engine to display the value you put in parenthesis on the console,
so that when you run console.log('Hello World!')
, the JavaScript engine will display the text Hello World!
In JavaScript, functions are a way to group code that can be reused or executed as many times as needed in a program.
With JavaScript functions, you can run the same set of statements from multiple places in a program without having to rewrite it each time.
This article addresses the following touchpoints:
- What functions are
- How to define functions in JavaScript
- How to pass arguments to functions
- How to return values from functions
- Function declarations and function expressions
- How to invoke JavaScript functions
- How to define Immediately invoked functions (IIFE)
How to define functions in JavaScript
JavaScript allows us to define functions in different ways. A common way to do this is through the function
keyword.
Let's write a function that multiplies any number by two and returns the result:
function multiplyByTwo(value) {
const result = value * 2
return result
}
From our above function;
- The name of our function is multiplyByTwo, which is provided right after the
function
keyword. - The parenthesis next to the function name holds the function parameters.
- Parameters are names used to represent the values we want to use in our functions.
- The code block after the opening curly brace is the function body.
- The
return
keyword is used to end the execution of a function while returning a specified value. This value is called the return value.
Function parameters
The parameters of a function are names used to represent real values we intend to provide when we call a function. In our example above, our function parameter is value
.
When we call the multiplyByTwo
function, we'll provide the actual value for this parameter.
What is a function call?
A function call or function invocation is a request to execute a function. We'll see how to do this when we use the multiplyByTwo
function.
The return keyword
We use the return
keyword to end the execution of a function while providing a specified value. In our case, the multiplyByTwo
function returns the calculation result.
Let's see how to use the return keyword to end the execution of our function conditionally:
// focus(2,3,4)
function multiplyByTwo(value) {
if (isNaN(value)) {
return ' Value must be a number'
}
const result = value * 2
return result
}
In our new function, we added a condition that checks if the value parameter is a number.
So, if the value
is not a number, the first return
statement would terminate the function and return the string, "Value must be a number".
The above method we used to define our JavaScript function is called function declaration. Now, let's tell our function to run the code it contains. We'll do this by invoking it. And to invoke our JavaScript function, we only need to write its name followed by a parenthesis:
multiplyByTwo()
Remember that in our function definition, we used the parameter, value
to represent the real value to be multiplied.
To provide that number to our function, we'll place it inside the parenthesis
invoking our function:
console.log(multiplyByTwo(35))
Here's what we'll see on our console when we run the above code:
70
Let's try supplying our function with a non-number:
console.log(multiplyByTwo('this is a string'))
Result:
Value must be a number
Function Parameters vs Arguments
When invoking our function, the values we supply to it in place of its parameters are known as arguments
.
So in our case, the number 35 and the string "this is a string" are our function arguments.
- During a function definition, the names representing the values we intend to supply to the function are called
parameters
.- During a function call, the actual we provide to the function are known as
arguments
.- We can use up to
255
parameters in a function definition.
More on the return statement
Without using the return
statement to tell our function what to return, its default value will be undefined
.
Let's test this by creating a function without a return
statement and then invoking it:
function multiplyByTwo(value) {
const result = value * 2
}
console.log(multiplyByTwo(35))
Result:
undefined
The function also returns undefined
by default when we provide the return
keyword without a value βthis makes it an empty return
:
// focus(3)
function multiplyByTwo() {
const result = value * 2
return
}
console.log(multiplyByTwo(35))
Result:
undefined
Anonymous functions
You've seen how to use function declarations to define named functions. You can also use function declarations to define anonymous functions and Immediately Invoked Function Expressions (IIFE).
Anonymous functions are functions that are without names; hence, the word anonymous.
function () {
return alert("I'm a function, and I'm anonymous!")
}
Since we cannot reference the above function by a name, there is no way to call it elsewhere in our codebase.
When this is the case, the function is usually an IIFE
(Immediately Invoked Function Expression), which means that it'll be invoked immediately after it is declared.
To achieve this, we'll wrap the function in parenthesis and immediately call it with another open-and-close parenthesis right after its declaration:
;(function () {
return alert("I'm a function, and I'm anonymous!")
})()
Notice how we declared our function inside the parenthesis before invoking it.
Function expression
To reuse an anonymous function, we have to assign it to a variable
. This way, we can reference it by that variable name
.
A way to do this is through Function expression. Let's assign our anonymous function to a variable:
const alertUser = function () {
return alert("I'm anonymous, and you can reference me by a name!")
}
Now, we can invoke (or call) our function the same way we invoked multiplyByTwo()
. Since our function has no parameter, there'll be no need to supply an argument.
alertUser()
Result:
I'm anonymous, and you can reference me by a name!
Multi-line return statements
When returning an expression in a function, don't add a new line between
return
and the value.
You might be tempted to separate your expression from the return
statement like this:
function reverseWord(word) {
return
word.split('').reverse().join('')
}
console.log(reverseWord('inevitable'))
Result:
undefined
Calling the above reverseWord
function will return undefined
, and this is because our compiler ends the statement by assuming a semicolon after the return
keyword, making it an empty return:
function reverseWord(word) {
return
word.split('').reverse().join('')
}
If you want to wrap the return statement across multiple lines, you should start your expression on the same line as the return
keyword or have an opening parenthesis on the same line as the return
keyword:
function reverseWord(word) {
return word.split('').reverse().join('')
}
console.log(reverseWord('inevitable'))
elbativeni
Or
function reverseWord(word) {
return word.split('').reverse().join('')
}
console.log(reverseWord('inevitable'))
elbativeni
Functions best practices
The name of a function should be descriptive, straight to the point and timeless.
Notice how we named our first function multiplyByTwo
. This is because its job is to multiply a number by two. Doing this will help anyone know what our function does by looking at its name.
Function naming is an essential part of function definition, and choosing to disregard this will make your code non-readable, non-maintainableand lead to reduced efficiency.
When naming your functions, it is essential not to use slangs that only a few people would understand or trends that people can only relate to at a given time.
Let your function names be descriptive enough, straight to the point and timeless.
A function should have only one responsibility.
When you define your function, let it have one responsibility and do only what its name suggests. This means that from our examples, choosing to reverse a word and multiply a number in the same function would not be a good idea. Like we did, splitting the two actions into separate functions is better.
By doing this, you would not only make your code more readable, but you'll also make it easier to test and debug.
Summary
- A function is a set of statements designed to perform a particular task.
- During a function definition, the names representing the values we intend to supply to the function are called
parameters
. - During a function call, the actual values provided to the function are called
arguments
. - We can use up to
255
parameters in a function definition. - An anonymous function is a function that does not have a name, and to reuse an anonymous function, we have to assign it to a variable.
- An
IIFE
(Immediately Invoked Function Expression) is a function that is invoked immediately after it is declared. - A function should have only one responsibility.
- Function names should be descriptive, straight to the point and timeless.
Thanks for reading! If you want to learn how to build mobile apps with only HTML, CSS and JavaScript, here's a link to my JavaScript Mobile App crash course.
Found this helpful? Please like this article π, comment π and share! π·