React vs Vue: Choosing the Right JavaScript Framework
May 25, 2023 • 0 minute read •
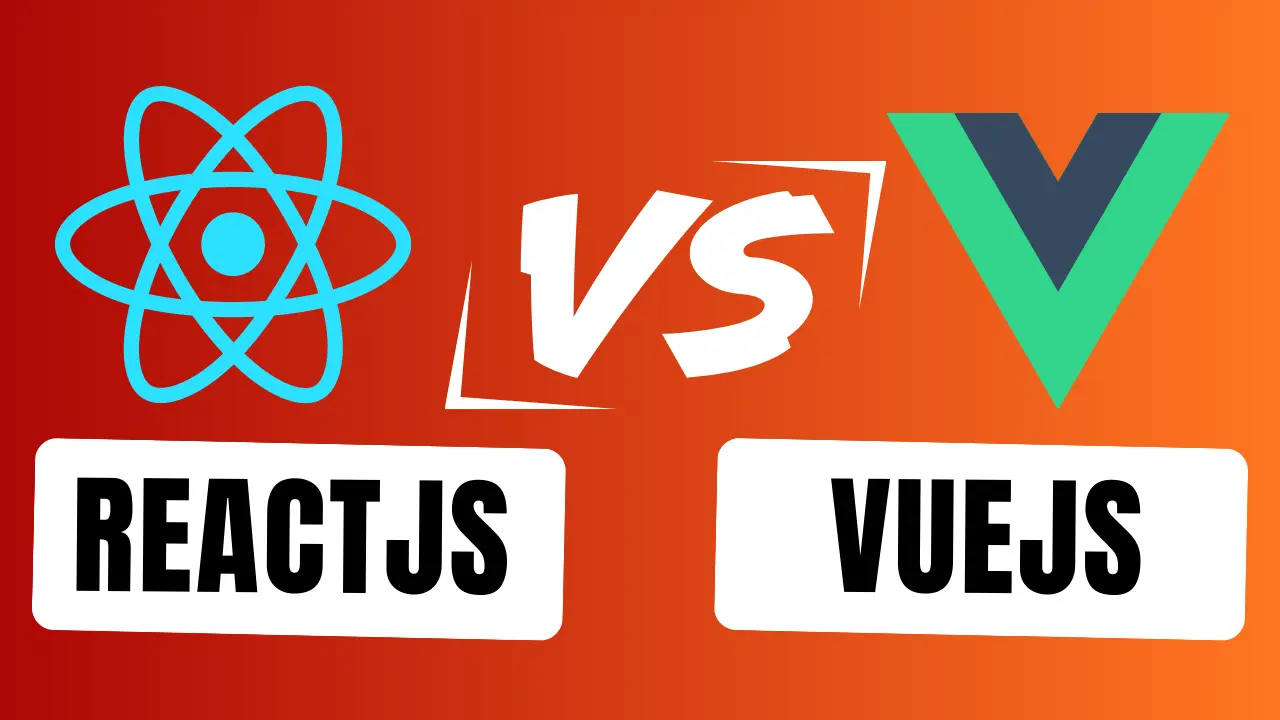
If you're venturing into frontend development, there's a good chance you've heard of ReactJS and VueJS and are probably wondering which one to learn. These JavaScript frameworks are popular choices for building user interfaces, and both have unique strengths. But before we explore their strengths and differences, let's take a step back and learn a bit about their history.
ReactJS: The library for User Interfaces
ReactJS, or simply React, is a creation of Facebook. In 2011, Facebook software engineer Jordan Walke designed a prototype to address the complexities of Facebook's user interfaces. The result was React—a JavaScript library that allows developers to create reusable UI components, making complex interfaces manageable.
React made its debut in the open-source community in 2013 and has been a prominent player in frontend development ever since.
VueJS: The Progressive JavaScript Framework
VueJS, or Vue for short, was developed by Evan You, an ex-Google engineer. After working with AngularJS at Google, Evan wanted to create a lighter, more flexible framework with a similar feature set. And so, in 2014, Vue was released. Its simplicity and flexibility quickly resonated with developers, and its popularity has been on the rise since its inception.
Vue, like React, is used for building user interfaces but has a different approach to component-based development.
In this article, we'll delve deeper into React and Vue. We'll examine their popularity and communities, learning curves, flexibility, job market demand, and performance. By the end, you'll better understand what each framework has to offer and be equipped to choose the one that best suits your needs. Ready to dive in? Let's get started!
Popularity and Community
The popularity of a framework and the strength of its community are important factors to consider. A larger community often means more learning resources, third-party libraries, and more help when you run into issues. So, how do React and Vue stack up?
ReactJS: A Strong Following
React has been around since 2013 and has a large, vibrant community. As of May 2023, React had over 200K stars on GitHub, a clear indication of its popularity.
This large community has produced a wealth of resources for learning React, including comprehensive documentation, tutorials, blog posts, and even dedicated online learning platforms. If you run into issues while working with React, chances are that someone else has encountered the same problem, and you'll find a solution on StackOverflow, GitHub, or other online forums.
The large community also means a vast ecosystem of third-party libraries to extend React's functionality. Libraries like Redux for state management, React Router for routing, and many others have been created by the community to solve common problems.
VueJS: Rapid Growth
Vue is a bit newer than React, released in 2014, but it has grown rapidly and built a strong community. Vue has different repositories for its version 2 and version 3 releases. As of May 2023, the version 2 repository had over 200k stars on GitHub, and version 3 had over 37k stars.
The Vue community is known for being friendly and welcoming, making it an excellent place for beginners. The official Vue documentation is highly praised for its clarity and thoroughness. In addition, plenty of Vue tutorials, courses, and blog posts are available to help you learn.
The Vue community has also created several useful libraries. Vuex for state management and Vue Router for routing are officially maintained and work seamlessly with Vue. Other third-party libraries are also available, although the ecosystem is less large than React's.
What Does This Mean For You?
Both React and Vue have large, active communities and many resources to help you learn. The choice between them may come down to personal preference.
If you prefer a mature community with a large ecosystem of third-party libraries, you might lean towards React. On the other hand, if you prefer a community known for its friendliness and excellent official resources, Vue may be the way to go.
Ultimately, both communities are passionate about their respective frameworks and committed to improving them. Whether you choose React or Vue, you'll join a group of developers eager to help and learn from each other.
Learning Curve
Now let's talk about how easy it is to pick up React and Vue. As a beginner, you want a framework with a gentle learning curve but also one capable enough to handle more advanced concepts as your skills develop. Both frameworks offer different experiences for beginners, so it's essential to consider the learning curve.
ReactJS: JavaScript, JSX, and Functional Components
React can initially feel a bit challenging, especially if you're new to JavaScript or programming in general. Here's an example of a simple functional component in React:
import React from 'react'
function Welcome(props) {
return <h1>Hello, {props.name}</h1>
}
export default Welcome
React uses a syntax extension called JSX, which combines JavaScript with HTML-like syntax. While JSX can make code more readable and writeable once you get the hang of it, it might initially seem foreign.
In addition, React leans heavily on understanding JavaScript and functional programming concepts. You'll need to get comfortable with JavaScript's ES6 features like arrow functions, destructuring, modules, and classes. And as you start building complex apps, you'll encounter advanced topics like state management with hooks, context, or third-party libraries like Redux.
VueJS: Template Syntax and Single-File Components
On the other hand, Vue tries to make the learning experience smoother for beginners. Here's a similar component as before but written in Vue:
<template>
<div>
<h1>Hello, {{ name }}</h1>
</div>
</template>
<script>
export default {
props: ['name'],
}
</script>
Vue uses an HTML-based template syntax that many find more intuitive and easier to pick up than JSX. It also introduces Single-File Components, where a component's template, script, and styles are contained within a single .vue
file. This approach makes it easier to understand and manage the various parts of a component.
While Vue also leverages modern JavaScript features, it tends to abstract away some of the complexity. This makes Vue easier to start with, especially for those with an HTML/CSS background. However, as you dive deeper into Vue, you will need to understand concepts like component lifecycle, directives, and advanced state management with Vuex.
Bottom Line: Learning Journey Matters
The choice between React and Vue can significantly depend on your comfort level with JavaScript, your background, and how you prefer to learn.
React might seem more challenging initially due to JSX and its heavy reliance on JavaScript concepts. But if you're keen on deep-diving into JavaScript and functional programming, you'll find React rewarding.
Vue, with its simpler syntax and more precise separation of concerns, offers a gentler start, especially if you're from an HTML/CSS background. It gradually introduces you to more advanced concepts, providing a more layered learning experience.
Remember, every developer's journey is unique, and there's no one-size-fits-all answer. Choose the framework that aligns with your learning style, goals, and project requirements. After all, the key to mastering any technology is consistent practice and patience!
Performance
In the world of web development, performance is paramount. Your users expect your applications to load quickly and run smoothly. So, how do React and Vue stack up in terms of performance? Both libraries have been optimized for high performance but handle rendering and updates differently.
ReactJS: Virtual DOM and Reconciliation
React uses a concept called the Virtual DOM (Document Object Model) to improve performance. The Virtual DOM is a lightweight copy of the actual DOM, allowing React to make updates efficiently. When a component's state changes, React creates a new Virtual DOM and compares it with the old one. This process is known as reconciliation.
Through reconciliation, React figures out the minimal number of operations needed to update the actual DOM to match the new Virtual DOM. This diffing process allows React to minimize expensive DOM operations, which results in improved performance.
However, this doesn't mean that React consistently outperforms other libraries. The performance of your React app can be influenced by many factors, including how you structure your components and manage state.
VueJS: Reactive Dependency Tracking
Vue also uses a Virtual DOM, but it employs a different strategy known as "reactive dependency tracking." This means that Vue tracks which components depend on which data, and when data changes, only the components that depend on that data are re-rendered.
Vue's reactivity system can make it more efficient than React in some instances, particularly when there are frequent updates to a small number of components. However, like React, the performance of your Vue app can be affected by your coding practices and application architecture.
Benchmarking Performance
While these theoretical differences are interesting, the real test is how React and Vue perform in real-world applications. Unfortunately, it's challenging to make direct comparisons because performance can be influenced by many factors, including the specific use case, the complexity of the app, and the developer's skill level.
However, according to benchmarking tests done using the JavaScript Framework Benchmark, React and Vue perform comparably well on most tasks. There are some tasks where Vue slightly outperforms React and vice versa. Still, the differences are generally not significant enough to be a deciding factor in choosing one over the other.
The Bottom Line
When it comes to performance, both React and Vue are designed to be highly performant and can handle most web development tasks with ease. Your choice between them might be based on other factors, such as your preference, the job market, and your specific project requirements.
Keep in mind that the most significant performance gains will likely come from good coding practices, efficient state management, and thoughtful application design rather than the choice of framework.
Flexibility
Flexibility is one of the most essential characteristics of any JavaScript framework. How much freedom does each framework give you to customize it to fit your specific needs, and how does that impact your work?
ReactJS: The Legos of JavaScript Frameworks
If you've ever played with Legos, you might recall starting with a bunch of individual pieces, and it's up to you to put them together to create your masterpiece. ReactJS can be considered the Lego blocks of the frontend world.
With React, you have the flexibility to choose and integrate the libraries that best suit your project. For instance, regarding state management, you could use Redux, Hookstate, or the Context API, each with its strengths and suitable use cases. This approach gives you the freedom to construct a unique solution that precisely meets your project requirements.
However, this flexibility is a double-edged sword. With so many choices, it can be overwhelming for beginners to make the right decisions, and integrating multiple libraries can lead to complexity and compatibility issues.
Imagine it's your first time playing with Legos, and you're given thousands of different pieces without instructions. It can be daunting and time-consuming to figure out where each piece goes. That's how it can feel to start a project in React without a good understanding of the ecosystem.
VueJS: The Pre-Built Model Kits
On the flip side, VueJS is more akin to a pre-built model kit. The creators have made certain choices for you and bundled in many solutions you would otherwise need to find on your own.
Vue comes with built-in solutions for things like state management and routing with Vuex and Vue Router, respectively. This opinionated nature means you can focus more on developing your application instead of deciding which libraries to use.
The primary benefit here is simplicity. It's like getting a model kit with instructions and all the pieces you need. This can be a blessing as a beginner, allowing you to get up and running more quickly without worrying about which pieces go where.
Yet, there's a trade-off: you might not have as much flexibility to replace these built-in pieces. However, Vue's design philosophy is such that it tries to balance flexibility and opinionatedness, which means you still have room for customization.
What Does this Mean for You?
So, which is better? As always, the answer depends on your needs and circumstances.
If you enjoy having complete control over your tech stack and don't mind the responsibility of choosing and integrating different libraries, you may find React more to your liking. It's like having a blank canvas where you can create your masterpiece with the Legos of your choice.
But if you prefer a framework that makes more decisions for you and provides a more streamlined setup, then Vue might be the way to go. It's like getting a model kit that's ready for assembly.
Ultimately, both React and Vue offer a balance between flexibility and ease of use. The right choice will depend on your project requirements, your comfort with JavaScript, and the time you are willing to invest in learning and decision-making. Remember, there's no "one size fits all" in the programming, and that's what makes it so exciting!
Job Market
When choosing a technology to learn, it's wise to consider the job market. After all, what's the use of mastering a skill if it isn't in demand? Fortunately, both ReactJS and VueJS are popular in the industry, but there are differences in their market share and the kinds of jobs available.
ReactJS: High Demand and Variety
As of my last update in September 2021, ReactJS consistently ranks as one of the most sought-after skills in job listings. Indeed.com, a popular job search site, often shows thousands of job listings requiring or preferring ReactJS skills.
React's wide usage in the industry is partially due to its adoption by large tech companies like Facebook, Instagram, and Netflix. This adoption by high-profile companies often leads to broader industry usage and, hence, greater demand in the job market.
VueJS: Growing Rapidly
VueJS, while not as popular as React in job postings, has seen considerable growth. The number of job listings seeking VueJS skills has increased steadily over the past few years.
Vue has been adopted by several high-profile companies like Alibaba, Xiaomi, and Adobe, which has helped increase its visibility and popularity in the job market. Though VueJS is a bit newer than React, it's been adopted widely, contributing to the growth in VueJS-related jobs.
So What Does this Mean for You?
Deciding which technology to learn based solely on job market trends can be risky. Technology is ever-evolving, and the demand for specific skills can change rapidly. However, both ReactJS and VueJS have significant industry adoption, which suggests they'll both continue to be in demand for the foreseeable future.
While React may offer more job opportunities right now, Vue is growing rapidly and could catch up. And remember, knowing more than one framework will only make you more versatile as a developer.
In the end, your choice should be guided by your interests and the kind of work you want to do. Both frameworks offer ample opportunities, so it's up to you to decide which path to follow.
Conclusion
And that's it for our comprehensive comparison between ReactJS and VueJS. As we've traversed through their communities, learning curves, flexibility, job market demand, and performance, you've probably noticed something: both are excellent choices.
React, with its robust community, vast ecosystem, and strong job market, is a great choice, especially if you're interested in diving deep into JavaScript. It's powerful, flexible, and well-suited for large-scale applications. Yet, remember that with React's flexibility comes the responsibility of making architectural decisions and integrating various libraries.
Vue, on the other hand, offers a more direct, intuitive learning experience, especially for those from an HTML/CSS background. Its built-in solutions, clear separation of concerns, and gentle learning curve make it an excellent option for beginners. However, its job market, while growing, isn't as large as React's.
Choosing between React and Vue will depend on many factors, including your comfort with JavaScript, project requirements, team preferences, and long-term career goals.
While making an informed decision is essential, remember that there's no absolute right or wrong choice. Both libraries will help you build impressive, high-performing web applications and, most importantly, make you a better developer.
So whether you choose to walk the path of React or journey down the Vue route, embrace the learning experience, and remember to enjoy the process. Because, after all, the tools we use are just a means to an end—the real magic lies in your creativity and code!
Love what you just read? Let me know in the comments section (coming soon!) or use the share button 💛 at the bottom-right to share this article with others. If you want to reach out to me directly, click on my NewDev profile to see all my social media links and contact info.
Heard of SolidJS? It's a new JavaScript library that's posed to replace React and Vue as the go-to frontend framework. Read about it here!